I'm using Python code with MSAL to read emails from my free Outlook account. I have set up Apps in Azure and correctly added permissions and granted admin access, etc. When I use the App that has delegated permissions using app.acquire_token_for_client it works as expected. However, when I use the App with application permissions using ConfidentialClientApplication, authentication fails. What could be the problem? Is it because I am using free Outlook and Azure accounts. All my research on-line indicates it should be possible.
The App permissions are as follows
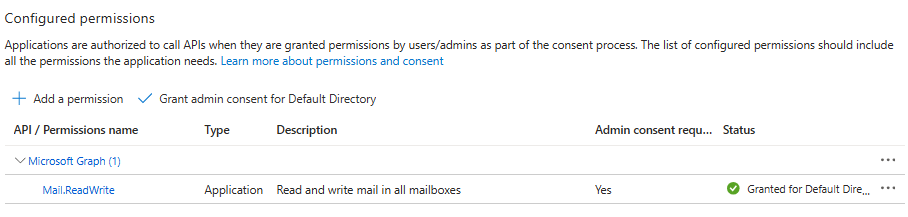
My Python code is below. When I run it I get:
Access token acquired successfully.
Failed to retrieve emails.
Status Code: 404
Response: {'error': {'code': 'ErrorInvalidUser', 'message': "The requested user '####@hotmail.com' is invalid."}}
from msal import ConfidentialClientApplication
import requests
# Replace with your own email address
user_email = "####@hotmail.com"
client_id = '#####################################'
client_secret = '###############################'
tenant_id = '###################################'
authority = f"https://login.microsoftonline.com/{tenant_id}"
# Acquire the access token
app = ConfidentialClientApplication(
client_id,
authority=authority,
client_credential=client_secret
)
scope = ["https://graph.microsoft.com/.default"]
result = app.acquire_token_for_client(scopes=scope)
if "access_token" in result:
access_token = result["access_token"]
print("Access token acquired successfully.")
else:
print("Failed to acquire token.")
print(result.get("error"))
print(result.get("error_description"))
print(result.get("correlation_id")) # You might need this when reporting a bug
exit()
# Make a request to the Microsoft Graph API to get the user's emails
headers = {
'Authorization': f'Bearer {access_token}',
'Content-Type': 'application/json'
}
# Correct the endpoint URL by using /users/{user_email}/mailFolders/inbox/messages
endpoint = f'https://graph.microsoft.com/v1.0/users/{user_email}/mailFolders/inbox/messages'
response = requests.get(endpoint, headers=headers)
if response.status_code == 200:
emails = response.json().get('value', [])
print(f"Found {len(emails)} emails in the inbox.")
for email in emails:
print(f"Subject: {email['subject']}")
print(f"From: {email['from']['emailAddress']['address']}")
print(f"Received: {email['receivedDateTime']}")
print("----")
else:
print("Failed to retrieve emails.")
print(f"Status Code: {response.status_code}")
print(f"Response: {response.json()}")