ID2D1RenderTarget::D rawRectangle(constD2D1_RECT_F&,ID2D1Brush*,FLOAT,ID2D1StrokeStyle*) 메서드(d2d1.h)
지정된 차원 및 스트로크 스타일이 있는 사각형의 윤곽선을 그립니다.
구문
void DrawRectangle(
const D2D1_RECT_F & rect,
ID2D1Brush *brush,
FLOAT strokeWidth,
ID2D1StrokeStyle *strokeStyle
);
매개 변수
rect
형식: [in] const D2D1_RECT_F &
그릴 사각형의 크기(디바이스 독립적 픽셀)입니다.
brush
형식: [in] ID2D1Brush*
직사각형의 스트로크를 그리는 데 사용되는 브러시입니다.
strokeWidth
형식: [in] FLOAT
스트로크의 너비(디바이스 독립적 픽셀)입니다. 값은 0.0f보다 크거나 같아야 합니다. 이 매개 변수를 지정하지 않으면 기본값은 1.0f입니다. 스트로크는 선 가운데에 있습니다.
strokeStyle
형식: [in, optional] ID2D1StrokeStyle*
칠할 스트로크 스타일 또는 단색 스트로크를 그리는 NULL 입니다.
반환 값
없음
설명
이 메서드가 실패하면 오류 코드를 반환하지 않습니다. 그리기 메서드(예: DrawRectangle)가 실패했는지 여부를 확인하려면 ID2D1RenderTarget::EndDraw 또는 ID2D1RenderTarget::Flush 메서드에서 반환된 결과를 검사.
예제
다음 예제에서는 ID2D1HwndRenderTarget 을 사용하여 여러 사각형을 그리고 채웁니다. 이 예제에서는 다음 그림에 표시된 출력을 생성합니다.
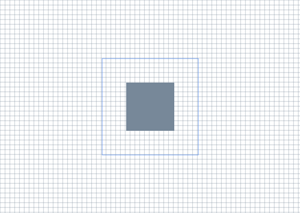
// This method discards device-specific
// resources if the Direct3D device disappears during execution and
// recreates the resources the next time it's invoked.
HRESULT DemoApp::OnRender()
{
HRESULT hr = S_OK;
hr = CreateDeviceResources();
if (SUCCEEDED(hr))
{
m_pRenderTarget->BeginDraw();
m_pRenderTarget->SetTransform(D2D1::Matrix3x2F::Identity());
m_pRenderTarget->Clear(D2D1::ColorF(D2D1::ColorF::White));
D2D1_SIZE_F rtSize = m_pRenderTarget->GetSize();
// Draw a grid background.
int width = static_cast<int>(rtSize.width);
int height = static_cast<int>(rtSize.height);
for (int x = 0; x < width; x += 10)
{
m_pRenderTarget->DrawLine(
D2D1::Point2F(static_cast<FLOAT>(x), 0.0f),
D2D1::Point2F(static_cast<FLOAT>(x), rtSize.height),
m_pLightSlateGrayBrush,
0.5f
);
}
for (int y = 0; y < height; y += 10)
{
m_pRenderTarget->DrawLine(
D2D1::Point2F(0.0f, static_cast<FLOAT>(y)),
D2D1::Point2F(rtSize.width, static_cast<FLOAT>(y)),
m_pLightSlateGrayBrush,
0.5f
);
}
// Draw two rectangles.
D2D1_RECT_F rectangle1 = D2D1::RectF(
rtSize.width/2 - 50.0f,
rtSize.height/2 - 50.0f,
rtSize.width/2 + 50.0f,
rtSize.height/2 + 50.0f
);
D2D1_RECT_F rectangle2 = D2D1::RectF(
rtSize.width/2 - 100.0f,
rtSize.height/2 - 100.0f,
rtSize.width/2 + 100.0f,
rtSize.height/2 + 100.0f
);
// Draw a filled rectangle.
m_pRenderTarget->FillRectangle(&rectangle1, m_pLightSlateGrayBrush);
// Draw the outline of a rectangle.
m_pRenderTarget->DrawRectangle(&rectangle2, m_pCornflowerBlueBrush);
hr = m_pRenderTarget->EndDraw();
}
if (hr == D2DERR_RECREATE_TARGET)
{
hr = S_OK;
DiscardDeviceResources();
}
return hr;
}
관련 자습서는 간단한 Direct2D 애플리케이션 만들기를 참조하세요.
요구 사항
요구 사항 | 값 |
---|---|
지원되는 최소 클라이언트 | Windows 7, Windows Vista SP2 및 Windows Vista용 플랫폼 업데이트 [데스크톱 앱 | UWP 앱] |
지원되는 최소 서버 | Windows Server 2008 R2, Windows Server 2008 SP2 및 Windows Server 2008용 플랫폼 업데이트 [데스크톱 앱 | UWP 앱] |
대상 플랫폼 | Windows |
헤더 | d2d1.h |
라이브러리 | D2d1.lib |
DLL | D2d1.dll |