ID2D1SolidColorBrush interface (d2d1.h)
Paints an area with a solid color.
Inheritance
The ID2D1SolidColorBrush interface inherits from ID2D1Brush. ID2D1SolidColorBrush also has these types of members:
Methods
The ID2D1SolidColorBrush interface has these methods.
ID2D1SolidColorBrush::GetColor Retrieves the color of the solid color brush. |
ID2D1SolidColorBrush::SetColor Specifies the color of this solid-color brush. |
ID2D1SolidColorBrush::SetColor Specifies the color of this solid color brush. |
Remarks
Creating ID2D1SolidColorBrush Objects
To create a solid color brush, use the ID2D1RenderTarget::CreateSolidColorBrush method of the render target on which the brush will be used. The brush can only be used with the render target that created it or with the compatible targets for that render target.
A solid color brush is a device-dependent resource. (For more information about resources, see Resources Overview.)
Examples
The following example uses the CreateSolidColorBrush method of a render target (m_pRenderTarget) to create two brushes. The example uses a predefined color (black) to specify the color of the first brush. It uses a hexadecimal color value (yellow) to specify the color of the second brush.
if (SUCCEEDED(hr))
{
hr = m_pRenderTarget->CreateSolidColorBrush(
D2D1::ColorF(D2D1::ColorF::Black, 1.0f),
&m_pBlackBrush
);
}
// Create a solid color brush with its rgb value 0x9ACD32.
if (SUCCEEDED(hr))
{
hr = m_pRenderTarget->CreateSolidColorBrush(
D2D1::ColorF(D2D1::ColorF(0x9ACD32, 1.0f)),
&m_pYellowGreenBrush
);
}
The next code example calls the FillRectangle method to paint the interior of a rectangle with the yellow green brush and the DrawRectangle method to paint the outline of the rectangle with the black brush:
m_pRenderTarget->FillRectangle(&rcBrushRect, m_pYellowGreenBrush);
m_pRenderTarget->DrawRectangle(&rcBrushRect, m_pBlackBrush, 1, NULL);
These examples produce the output shown in the following illustration.
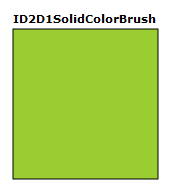
Requirements
Requirement | Value |
---|---|
Minimum supported client | Windows 7, Windows Vista with SP2 and Platform Update for Windows Vista [desktop apps | UWP apps] |
Minimum supported server | Windows Server 2008 R2, Windows Server 2008 with SP2 and Platform Update for Windows Server 2008 [desktop apps | UWP apps] |
Target Platform | Windows |
Header | d2d1.h |
See also
Feedback
https://aka.ms/ContentUserFeedback.
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see:Submit and view feedback for