Método ID2D1RenderTarget::D rawRectangle(constD2D1_RECT_F&,ID2D1Brush*,FLOAT,ID2D1StrokeStyle*) (d2d1.h)
Desenha a estrutura de tópicos de um retângulo que tem as dimensões e o estilo de traço especificados.
Sintaxe
void DrawRectangle(
const D2D1_RECT_F & rect,
ID2D1Brush *brush,
FLOAT strokeWidth,
ID2D1StrokeStyle *strokeStyle
);
Parâmetros
rect
Tipo: [in] const D2D1_RECT_F &
As dimensões do retângulo a ser desenhado, em pixels independentes do dispositivo.
brush
Tipo: [in] ID2D1Brush*
O pincel usado para pintar o traço do retângulo.
strokeWidth
Tipo: [in] FLOAT
A largura do traço, em pixels independentes do dispositivo. O valor deve ser maior ou igual a 0,0f. Se esse parâmetro não for especificado, o padrão será 1.0f. O traço está centralizado na linha.
strokeStyle
Tipo: [in, opcional] ID2D1StrokeStyle*
O estilo de traço a ser pintado ou NULL para pintar um traço sólido.
Retornar valor
Nenhum
Comentários
Quando esse método falha, ele não retorna um código de erro. Para determinar se um método de desenho (como DrawRectangle) falhou, marcar o resultado retornado pelo método ID2D1RenderTarget::EndDraw ou ID2D1RenderTarget::Flush.
Exemplos
O exemplo a seguir usa um ID2D1HwndRenderTarget para desenhar e preencher vários retângulos. Este exemplo produz a saída mostrada na ilustração a seguir.
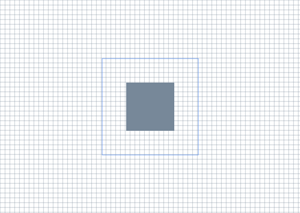
// This method discards device-specific
// resources if the Direct3D device disappears during execution and
// recreates the resources the next time it's invoked.
HRESULT DemoApp::OnRender()
{
HRESULT hr = S_OK;
hr = CreateDeviceResources();
if (SUCCEEDED(hr))
{
m_pRenderTarget->BeginDraw();
m_pRenderTarget->SetTransform(D2D1::Matrix3x2F::Identity());
m_pRenderTarget->Clear(D2D1::ColorF(D2D1::ColorF::White));
D2D1_SIZE_F rtSize = m_pRenderTarget->GetSize();
// Draw a grid background.
int width = static_cast<int>(rtSize.width);
int height = static_cast<int>(rtSize.height);
for (int x = 0; x < width; x += 10)
{
m_pRenderTarget->DrawLine(
D2D1::Point2F(static_cast<FLOAT>(x), 0.0f),
D2D1::Point2F(static_cast<FLOAT>(x), rtSize.height),
m_pLightSlateGrayBrush,
0.5f
);
}
for (int y = 0; y < height; y += 10)
{
m_pRenderTarget->DrawLine(
D2D1::Point2F(0.0f, static_cast<FLOAT>(y)),
D2D1::Point2F(rtSize.width, static_cast<FLOAT>(y)),
m_pLightSlateGrayBrush,
0.5f
);
}
// Draw two rectangles.
D2D1_RECT_F rectangle1 = D2D1::RectF(
rtSize.width/2 - 50.0f,
rtSize.height/2 - 50.0f,
rtSize.width/2 + 50.0f,
rtSize.height/2 + 50.0f
);
D2D1_RECT_F rectangle2 = D2D1::RectF(
rtSize.width/2 - 100.0f,
rtSize.height/2 - 100.0f,
rtSize.width/2 + 100.0f,
rtSize.height/2 + 100.0f
);
// Draw a filled rectangle.
m_pRenderTarget->FillRectangle(&rectangle1, m_pLightSlateGrayBrush);
// Draw the outline of a rectangle.
m_pRenderTarget->DrawRectangle(&rectangle2, m_pCornflowerBlueBrush);
hr = m_pRenderTarget->EndDraw();
}
if (hr == D2DERR_RECREATE_TARGET)
{
hr = S_OK;
DiscardDeviceResources();
}
return hr;
}
Para obter um tutorial relacionado, consulte Criar um aplicativo de Direct2D simples.
Requisitos
Requisito | Valor |
---|---|
Cliente mínimo com suporte | Windows 7, Windows Vista com SP2 e Atualização de Plataforma para Windows Vista [aplicativos da área de trabalho | Aplicativos UWP] |
Servidor mínimo com suporte | Windows Server 2008 R2, Windows Server 2008 com SP2 e Atualização de Plataforma para Windows Server 2008 [aplicativos da área de trabalho | Aplicativos UWP] |
Plataforma de Destino | Windows |
Cabeçalho | d2d1.h |
Biblioteca | D2d1.lib |
DLL | D2d1.dll |
Confira também
Criar um aplicativo Direct2D simples
Comentários
https://aka.ms/ContentUserFeedback.
Em breve: Ao longo de 2024, eliminaremos os problemas do GitHub como o mecanismo de comentários para conteúdo e o substituiremos por um novo sistema de comentários. Para obter mais informações, consulteEnviar e exibir comentários de